本文分享的技术,是用Web做一个二分查找算法的小网页,包括所有的代码。通过二分查找的原理,做一个闭环Web小应用,这个网页包括HTML、CSS和JavaScript的配合。通过制作这个网页,老铁们可以熟悉网页设计、练习算法的落地应用,做到学以致用。
最终的二分算法网页效果展示
本文代码分为3个部分:核心JavaScript代码讲解(包括调用函数JS代码);
HTML网页设计讲解;
CSS的美化讲解。
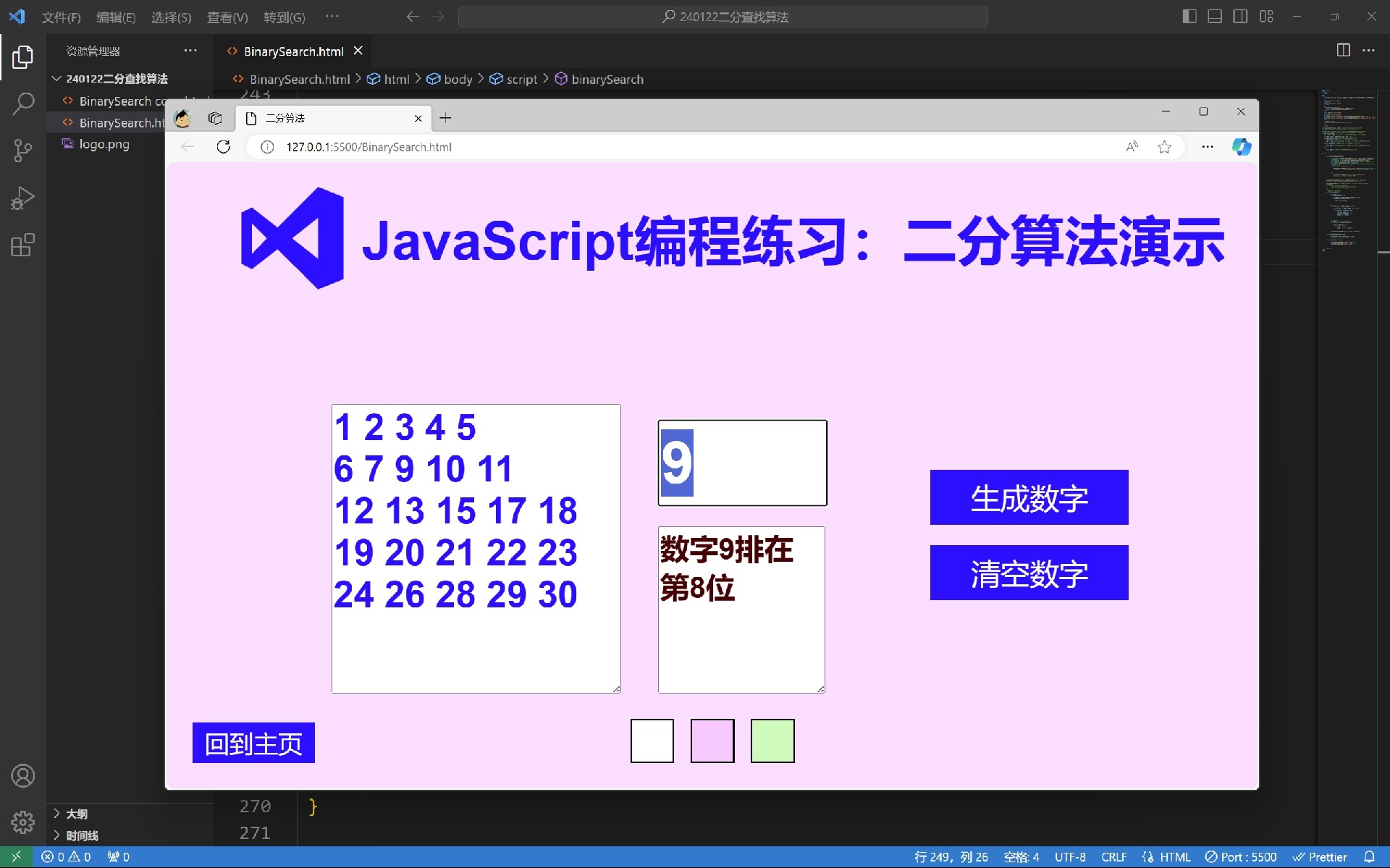
第1部分 JavaScript代码讲解
本案例的JavaScript代码包括核心的算法代码和调用代码。
先介绍核心算法代码:
function binarySearch(nums, target) //定义了一个名为 binarySearch 的函数, //该函数接受两个参数,nums 是已排序数组,target 是要查找的目标值。 { let left = 0;//声明两个变量 left 和 right,它们分别代表搜索范围的左边界和右边界。 //初始时,左边界为数组的第一个元素索引(0),右边界为数组的最后一个元素索引。 let right = nums.length - 1; while (left <= right) //使用循环来执行二分查找,条件是左边界小于等于右边界。 { let middle = left + Math.floor((right - left) / 2); //计算当前搜索范围的中间位置,使用 Math.floor 函数确保取整。 if (nums[middle] > target) //如果中间位置的元素值大于目标值。 { right = middle - 1;//将右边界更新为中间位置的前一个位置,缩小搜索范围至左半部分。 } else if (nums[middle] < target) //如果中间位置的元素值小于目标值。 { left = middle + 1;//将左边界更新为中间位置的后一个位置,缩小搜索范围至右半部分。 } else { return middle;//直接返回中间位置的索引,表示找到目标值。 } } return -1; }
这段代码的含义是:先定义两个边界索引,左边界初始值为0,右边界初始值为数组的长度。循环条件是左边界的数值小于右边界。
二分查找原理演示
二分查找是很基础的算法,很容易搜索到,在此不做更多的原理讲解。只要记住查找的数组必须有序排列即可。
由于这个算法要在Web端调用,因此还需要进行调用代码的编写:
function calculateBinarySearch() { const inputNums = document.getElementById("output").value.split(" ").map(Number); // 获取页面上 id 为 "output" 的元素的值,将其以空格分割成数字数组并映射为数字类型 const inputTarget = parseInt(document.getElementById("textBox1").value); // 获取页面上 id 为 "textBox1" 的元素的值,将其解析为整数,作为二分查找的目标值 const result = binarySearch(inputNums, inputTarget); // 调用二分查找函数 binarySearch,传入数字数组和目标值,并将结果保存在变量 result 中 if (result !== -1) // 如果结果不等于 -1,表示找到了目标值 { document.getElementById("textBox2").value = `数字${inputTarget}排在第${result + 1}位`; // 在页面上 id 为 "textBox2" 的元素中显示目标值在数组中的位置(索引 + 1) } else { document.getElementById("textBox2").value = "这个数字不存在"; // 如果结果等于 -1,表示目标值不存在于数组中 } }
在本案例中,显示25个数字的窗口是一个文本框“output”,一个输入查找数字的文本框“textBox1”和一个输出查找结果的文本框“textBox2”。
调用代码是将随机生成的25个数字提取成数组,把textBox1里输入的数字也进行必要的清洗,然后作为二分查找函数的输入值,进行计算,结算结果显示在textBox2中。显示结果要进行一下必要的美化,做一下人机交互的设计。
如何生成25个随机数字,我会在另一篇文章仔细讲解,在此不做赘述。
第2部分 HTML代码讲解
本案例的HTML包括一个标题文件(其中包含一个LOGO图片)、1个展示25个数字的文本框output,1个输入文本框textBox1和1个输出文本框textBox2。还有两个按钮,分别控制生成25个数字和清除25个数字。
<h1> <img src="logo.png" alt="Logo" width="130" height="130">JavaScript编程练习:二分算法演示 </h1> <textarea id="output" readonly> </textarea> <textarea id="textBox2" readonly> </textarea> <br> <div class="button-container"> <button onclick="generateNumbers()">生成数字</button> <button onclick="clearText()">清空数字</button> </div> <input type="text" id="textBox1"> <div class="color-box-container"> <div class="color-box" id="whiteBox" onclick="changeBackgroundColor('white')"></div> <div class="color-box" id="blueBox" onclick="changeBackgroundColor('rgba(247, 160, 255, 0.348)')"></div> <div class="color-box" id="pinkBox" onclick="changeBackgroundColor('rgba(202, 251, 189, 0.966)')"></div> </div> <a href="index.html"> <button id="backToHomeButton">回到主页</button> </a>
第3部分 CSS代码讲解
CSS代码考虑到了手机竖屏的应用。注意按钮使用了群组,本案例还有颜色代码块,纯装饰用,不是必须的。
<head> <meta name="viewport" content="width=device-width, initial-scale=0.5"> <meta charset="UTF-8"> <title>二分算法</title> <style> @media screen and (max-width: 768px) { #output { position: static; width: 80%; /* 调整文本框宽度 */ top:20%; text-align: left; } .button-container { position: fixed; margin-top: 20px; /* 调整按钮与文本框的间距 */ display: flex; flex-direction: column; align-items: left; } #textBox1 { position: static; width: 80%; /* Adjust the width as needed */ margin-top: 20px; } #textBox2 { position: static; width: 80%; /* Adjust the width as needed */ margin-top: 50px; } } #output/*输出的文本框格式*/ { width: 350px; height: 350px; font-size: 45px; font-family: Arial, Helvetica, sans-serif; color: blue; font-weight: bold; position: fixed; bottom: 15%; left: 15%; display: flex; } #textBox1 { width: 200px; height: 100px; font-size: 72px; font-family: Arial, Helvetica, sans-serif; color: rgb(74, 0, 0); font-weight: bold; position: fixed; bottom: 45%; left: 45%; display: flex; } #textBox2 { width: 200px; height: 200px; font-size: 36px; font-family: Arial, Helvetica, sans-serif; color:rgb(74, 0, 0); font-weight: bold; position: fixed; bottom: 15%; left: 45%; display: flex; } #textBox1::placeholder { font-size: 32px; /* 或者可以使用具体的数值,例如 font-size: 12px; */ vertical-align: middle; } .button-container /*按钮群组,不用群组不好管理*/ { position: fixed; bottom: 30%; left: 70%; display: flex; flex-direction: column; } body /*主题格式*/ { display: flex; flex-direction: column; align-items: center; font-family: Arial, sans-serif; } h1 /*标题*/ { display: flex; font-size: 66px; color: blue; margin-top: 20px; align-items: center; } img /*图片通用格式*/ { margin-right: 20px; margin-left: 50px; } button { margin-top: 25px; margin-right: 25px; font-size: 36px; color: white; background-color: blue; border: none; padding: 10px 50px; cursor: pointer; } button:hover { background-color: #2980b9; } #bottomRightImage { position: fixed; bottom: 10px; right: 10px; margin: 10px; width: auto; height: 10%; } #bottomLeftImage { position: fixed; bottom: 0px; left: 0px; margin: 0px; width: auto; height: 50%; } .color-box-container { position:fixed; bottom:0; margin-bottom: 20px; align-items: center; display: flex; } .color-box { width: 50px; height: 50px; margin: 10px; border: 2px solid black; cursor: pointer; } #whiteBox { background-color: white; } #blueBox { background-color: rgba(247, 160, 255, 0.348); } #pinkBox { background-color: rgba(202, 251, 189, 0.966); } #backToHomeButton { height: 50px; position:fixed; bottom:0; margin-bottom: 30px; left: 30px; font-size:30px; color:white; background-color: blue; border:none; padding:5px 15px; cursor:pointer; } #backToHomeButton:hover { background-color: #2980b9; } </style> </head>
宇哥课程广告
(长按二维码进入店铺,了解Access课程目录和介绍,点击订阅直接购买并观看录播课)
![]() | ![]() | ![]() |
![]() |
有详细问题请加宇哥微信:datamap1999